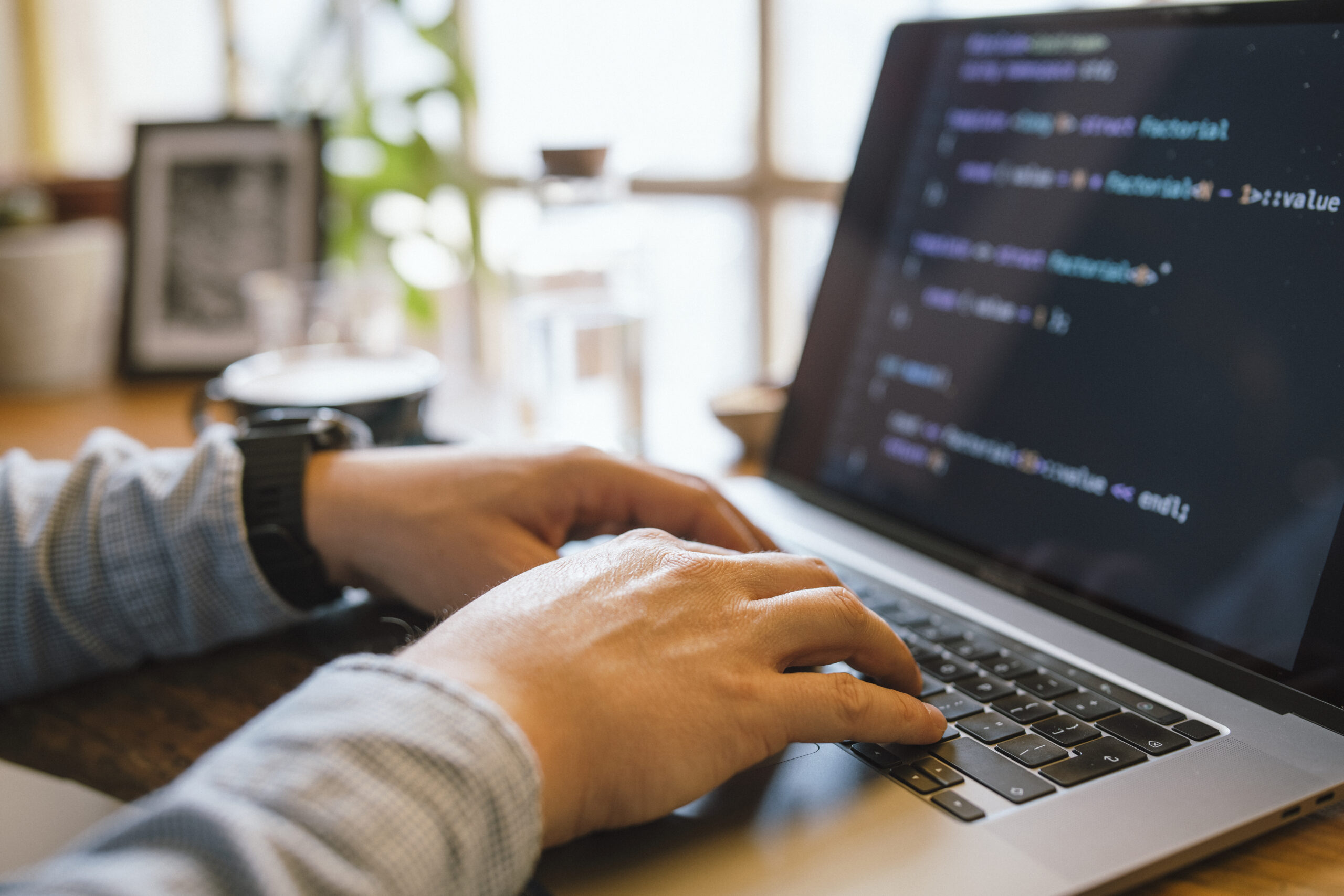
Debugging is One of the more crucial — still often ignored — expertise in the developer’s toolkit. It's actually not pretty much fixing damaged code; it’s about comprehending how and why points go Completely wrong, and learning to Believe methodically to solve issues effectively. No matter if you are a rookie or maybe a seasoned developer, sharpening your debugging techniques can help save hrs of frustration and dramatically improve your productivity. Here are several procedures to aid developers level up their debugging activity by me, Gustavo Woltmann.
Learn Your Instruments
Among the list of quickest strategies builders can elevate their debugging expertise is by mastering the tools they use every day. Though producing code is a person Component of growth, realizing how you can connect with it properly through execution is equally essential. Fashionable growth environments arrive Geared up with impressive debugging capabilities — but many builders only scratch the surface area of what these instruments can do.
Take, for instance, an Built-in Progress Surroundings (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These instruments let you established breakpoints, inspect the value of variables at runtime, action by means of code line by line, and even modify code to the fly. When utilised the right way, they Enable you to observe accurately how your code behaves through execution, and that is invaluable for monitoring down elusive bugs.
Browser developer equipment, such as Chrome DevTools, are indispensable for front-finish builders. They enable you to inspect the DOM, check network requests, view true-time overall performance metrics, and debug JavaScript during the browser. Mastering the console, sources, and network tabs can switch aggravating UI challenges into workable tasks.
For backend or technique-amount builders, equipment like GDB (GNU Debugger), Valgrind, or LLDB provide deep control in excess of operating processes and memory administration. Studying these applications can have a steeper Finding out curve but pays off when debugging performance problems, memory leaks, or segmentation faults.
Outside of your IDE or debugger, come to be comfy with Model Manage devices like Git to be aware of code record, locate the precise moment bugs were being introduced, and isolate problematic variations.
In the long run, mastering your equipment means likely past default options and shortcuts — it’s about producing an personal understanding of your progress ecosystem to ensure that when problems come up, you’re not misplaced in the dead of night. The greater you already know your applications, the greater time you may shell out resolving the particular trouble rather then fumbling as a result of the procedure.
Reproduce the situation
Among the most important — and often overlooked — ways in helpful debugging is reproducing the situation. In advance of jumping in to the code or generating guesses, developers need to produce a dependable natural environment or circumstance in which the bug reliably appears. With out reproducibility, correcting a bug will become a match of opportunity, often bringing about squandered time and fragile code alterations.
The first step in reproducing a dilemma is collecting as much context as is possible. Request queries like: What steps brought about the issue? Which natural environment was it in — advancement, staging, or production? Are there any logs, screenshots, or mistake messages? The more element you may have, the less difficult it gets to be to isolate the precise circumstances less than which the bug occurs.
After you’ve gathered adequate information, try and recreate the issue in your neighborhood atmosphere. This could indicate inputting the same knowledge, simulating similar consumer interactions, or mimicking procedure states. If the issue seems intermittently, contemplate crafting automated assessments that replicate the sting circumstances or point out transitions involved. These assessments not only support expose the problem but in addition reduce regressions in the future.
Often, the issue could possibly be ecosystem-particular — it would transpire only on certain working units, browsers, or under certain configurations. Working with applications like virtual machines, containerization (e.g., Docker), or cross-browser testing platforms might be instrumental in replicating this kind of bugs.
Reproducing the trouble isn’t merely a action — it’s a mindset. It demands persistence, observation, and a methodical approach. But after you can persistently recreate the bug, you might be now midway to fixing it. With a reproducible scenario, You should utilize your debugging applications extra correctly, exam potential fixes properly, and connect extra Obviously together with your group or consumers. It turns an abstract complaint right into a concrete obstacle — Which’s where developers prosper.
Browse and Have an understanding of the Mistake Messages
Mistake messages are sometimes the most beneficial clues a developer has when a little something goes Completely wrong. Rather than looking at them as discouraging interruptions, developers must discover to treat mistake messages as immediate communications through the program. They frequently show you what precisely took place, where by it took place, and at times even why it happened — if you know the way to interpret them.
Commence by studying the information meticulously As well as in total. Many builders, especially when less than time strain, glance at the 1st line and straight away start off producing assumptions. But further inside the mistake stack or logs may possibly lie the accurate root induce. Don’t just duplicate and paste error messages into search engines — examine and comprehend them to start with.
Break the mistake down into components. Is it a syntax error, a runtime exception, or a logic error? Will it stage to a selected file and line quantity? What module or purpose activated it? These questions can information your investigation and point you toward the liable code.
It’s also useful to be aware of the terminology of the programming language or framework you’re employing. Error messages in languages like Python, JavaScript, or Java generally stick to predictable designs, and learning to recognize these can considerably speed up your debugging method.
Some mistakes are obscure or generic, As well as in those circumstances, it’s important to look at the context by which the error transpired. Verify relevant log entries, enter values, and up to date changes inside the codebase.
Don’t forget compiler or linter warnings possibly. These often precede much larger challenges and provide hints about possible bugs.
Eventually, mistake messages are certainly not your enemies—they’re your guides. Learning to interpret them properly turns chaos into clarity, aiding you pinpoint challenges faster, minimize debugging time, and become a far more successful and self-confident developer.
Use Logging Sensibly
Logging is Just about the most impressive tools in a developer’s debugging toolkit. When utilized successfully, it provides genuine-time insights into how an application behaves, assisting you comprehend what’s occurring beneath the hood with no need to pause execution or phase throughout the code line by line.
An excellent logging method begins with realizing what to log and at what degree. Prevalent logging stages incorporate DEBUG, Data, WARN, ERROR, and Lethal. Use DEBUG for specific diagnostic facts for the duration of enhancement, Facts for normal gatherings (like prosperous start off-ups), WARN for potential challenges that don’t crack the applying, ERROR for real problems, and Lethal once the method can’t go on.
Prevent flooding your logs with abnormal or irrelevant facts. A lot of logging can obscure essential messages and decelerate your technique. Concentrate on key gatherings, state variations, enter/output values, and significant choice details within your code.
Structure your log messages Obviously and consistently. Include things like context, including timestamps, request IDs, and performance names, so it’s simpler to trace issues in distributed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs let you observe how variables evolve, what ailments are met, and what branches of logic are executed—all devoid of halting the program. They’re Specially valuable in generation environments where stepping by way of code isn’t possible.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about stability and clarity. That has a well-imagined-out logging solution, it is possible to lessen the time it will take to identify challenges, acquire deeper visibility into your apps, and Increase the General maintainability and dependability of your code.
Consider Similar to a Detective
Debugging is not just a technical activity—it is a method of investigation. To successfully recognize and deal with bugs, developers need to technique the procedure similar to a detective resolving a secret. This state of mind aids break down intricate issues into manageable components and stick to clues logically to uncover the basis lead to.
Start out by accumulating proof. Think about the indications of the issue: error messages, incorrect output, or efficiency concerns. Similar to a detective surveys a criminal offense scene, acquire as much pertinent details as it is possible to with out jumping to conclusions. Use logs, test instances, and user reports to piece alongside one another a transparent photo of what’s occurring.
Following, variety hypotheses. Talk to on your own: What may very well be resulting in this habits? Have any adjustments not too long ago been produced towards the codebase? Has this issue occurred before less than very similar situation? The aim would be to slender down options and discover prospective culprits.
Then, test your theories systematically. Attempt to recreate the problem inside of a controlled atmosphere. If you suspect a certain perform or ingredient, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, talk to your code inquiries and Allow the results guide you closer to the reality.
Pay out shut consideration to little specifics. Bugs frequently disguise while in the least predicted locations—similar to a missing semicolon, an off-by-one mistake, or perhaps a race issue. Be thorough and individual, resisting the urge to patch the issue with no fully knowledge it. Temporary here fixes may well hide the true problem, just for it to resurface later.
And finally, continue to keep notes on Whatever you tried using and discovered. Equally as detectives log their investigations, documenting your debugging system can conserve time for long run problems and support Other individuals have an understanding of your reasoning.
By considering just like a detective, builders can sharpen their analytical skills, strategy challenges methodically, and become more effective at uncovering hidden difficulties in sophisticated devices.
Generate Tests
Creating assessments is among the simplest tips on how to enhance your debugging expertise and Total enhancement effectiveness. Assessments not simply assistance capture bugs early but also serve as a safety net that gives you self-assurance when generating improvements to your codebase. A nicely-tested application is easier to debug because it enables you to pinpoint specifically in which and when a difficulty happens.
Start with unit assessments, which target particular person features or modules. These modest, isolated assessments can swiftly reveal regardless of whether a particular piece of logic is working as expected. Whenever a check fails, you instantly know where to look, significantly lessening enough time put in debugging. Unit tests are Primarily practical for catching regression bugs—problems that reappear after Beforehand currently being mounted.
Subsequent, combine integration assessments and stop-to-finish checks into your workflow. These enable be certain that different areas of your application do the job collectively easily. They’re significantly handy for catching bugs that take place in complex devices with several components or expert services interacting. If a little something breaks, your exams can tell you which Component of the pipeline failed and underneath what situations.
Crafting exams also forces you to definitely Consider critically regarding your code. To test a aspect appropriately, you may need to know its inputs, predicted outputs, and edge instances. This standard of comprehending Obviously prospects to raised code structure and less bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug could be a robust first step. After the exam fails regularly, you may focus on repairing the bug and enjoy your test move when The difficulty is fixed. This method makes certain that the identical bug doesn’t return Sooner or later.
In short, creating assessments turns debugging from the disheartening guessing sport into a structured and predictable course of action—encouraging you catch a lot more bugs, quicker and a lot more reliably.
Acquire Breaks
When debugging a tough issue, it’s simple to become immersed in the challenge—observing your monitor for several hours, trying Answer right after Resolution. But Among the most underrated debugging tools is simply stepping away. Taking breaks assists you reset your thoughts, minimize stress, and sometimes see The problem from a new viewpoint.
When you're as well close to the code for way too very long, cognitive tiredness sets in. You would possibly start out overlooking evident glitches or misreading code that you just wrote just hrs previously. On this state, your brain results in being fewer economical at problem-resolving. A brief stroll, a coffee break, or simply switching to another undertaking for 10–15 minutes can refresh your focus. Lots of builders report obtaining the basis of a challenge once they've taken time for you to disconnect, permitting their subconscious do the job while in the track record.
Breaks also assist prevent burnout, In particular for the duration of for a longer time debugging sessions. Sitting down in front of a monitor, mentally caught, is not simply unproductive but in addition draining. Stepping absent means that you can return with renewed Vitality along with a clearer mentality. You could possibly all of a sudden see a missing semicolon, a logic flaw, or a misplaced variable that eluded you in advance of.
Should you’re trapped, an excellent general guideline should be to set a timer—debug actively for forty five–60 minutes, then have a 5–ten moment split. Use that point to move all over, stretch, or do a thing unrelated to code. It may sense counterintuitive, Particularly under restricted deadlines, but it in fact leads to more rapidly and more practical debugging Over time.
To put it briefly, using breaks will not be a sign of weakness—it’s a wise strategy. It provides your Mind Area to breathe, increases your perspective, and will help you steer clear of the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and rest is an element of resolving it.
Discover From Every single Bug
Every bug you experience is much more than simply a temporary setback—It truly is a possibility to grow as being a developer. No matter whether it’s a syntax mistake, a logic flaw, or simply a deep architectural problem, each can train you a thing important in the event you take some time to mirror and assess what went Completely wrong.
Start by asking by yourself some key questions once the bug is resolved: What triggered it? Why did it go unnoticed? Could it have already been caught previously with greater techniques like device screening, code testimonials, or logging? The solutions typically expose blind spots as part of your workflow or being familiar with and assist you build much better coding habits going ahead.
Documenting bugs can even be an outstanding practice. Retain a developer journal or retain a log in which you Notice down bugs you’ve encountered, how you solved them, and what you discovered. As time passes, you’ll start to see patterns—recurring issues or common issues—you can proactively prevent.
In crew environments, sharing Everything you've discovered from the bug with the peers may be Primarily highly effective. No matter if it’s by way of a Slack message, a brief compose-up, or A fast knowledge-sharing session, encouraging Other folks avoid the exact challenge boosts group performance and cultivates a more robust Studying society.
Far more importantly, viewing bugs as lessons shifts your way of thinking from disappointment to curiosity. Instead of dreading bugs, you’ll start out appreciating them as crucial aspects of your growth journey. In the end, many of the finest developers will not be the ones who publish perfect code, but individuals who continuously understand from their mistakes.
In the long run, each bug you correct provides a fresh layer towards your skill set. So future time you squash a bug, take a minute to replicate—you’ll appear absent a smarter, much more capable developer thanks to it.
Conclusion
Strengthening your debugging skills will take time, exercise, and patience — nevertheless the payoff is big. It makes you a more productive, self-assured, and able developer. The next time you are knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be much better at Whatever you do.